Good Debugging Practices
For this week's devlog, we'll be giving tips for debugging your code in Gamemaker Studio.
Comments
Comments are pieces of text in a code file that are not compiled when the game is run. They are denoted as follows:
// Single line comment
/* Multi-Line Comment */
Commenting your code is the single most helpful thing you can do when both coding and debugging your code. It allows both you and your fellow teammates to more easily revisit and understand your code. It is extremely useful tagging lines or segments of code to describe their purpose. I suggest:
Labeling Methods - Methods are used to abstract portions of repeated code. Using comments to describe each method's intent or function can help you realize it is not working properly or make it easier for your teammates to understand its function.
Describing If-Statements - Describing what each if-statement's condition is can lead makes many nested or sequential if-statements far more readable
End Brackets - It is easy to tangle up your end brackets causing code to run or not run when you want it to. Adding simple comments describing want is ending can help you straighten this out
Naming Conventions
When naming objects or variables, give them names that make sense and properly explain their purpose. Don’t label them with a random letter or a name that’s completely unrelated to its purpose.
Ex: Health for a player
- Good: pHealth, playerHealth, health
- Bad: h, p, supercalifragilisticexpialidocious
Don’t forget that variables are case sensitive, “health” and “Health” are two different variables even if they are technically the same name. It's also good practice to prefix your assets with the type of asset it is, i.e. obj_player for a player object, or spr_player for a player sprite. This way, it's easier to identify which asset is which.
Print Statements
If you're finding run-time errors in your code, it can be helpful to use print statements to see the 'behind the scenes' of your game. Here are some of Gamemaker's built-in debug statements:
show_debug_message(string); //the most general print statement in GMS. If you want to print variables, concatenate your variables with a string; i.e. “string” + variable
show_debug_message("Player speed " + p_speed);
print(string); //functions just like show_debug_message, except variables are automatically casted to strings
print("Player Speed ", p_speed);
print_start( ); print_end( ); //both these print statements are used to separate your debug messages. This is simply to separate your messages so it’s more readable.
print_start("Player"); print("speed ", p_speed, " health ", p_health); print_end();
Gamemaker has more specific debug statements as well, but you should only need the ones above to help effectively debug your code.
Rule 3
Rule 3 is my personal favorite of the debuggin rules - When in doubt, do something stupid.
In more complex terms, whenever you can’t discern what is causing your bug, do something ridiculous. This most often involves setting variables to incredibly large or small values or changing an objects initialization location to inside a wall.
Observing how these situations effect the outcome of your game can provide you with clues as to why your genuine code is not functioning as it should. Especially when dealing with things like movement, damage, or combat.
Rubber Duck Debugging
Another way to debug your code is to explain your code line by line to someone or something else. This is called rubber duck debugging, which is a reference to a programmer that would carry around a rubber duck and force themselves to explain their code to the duck while debugging. Verbally explaining your code to yourself or a group member can be a great way to find any bugs and improve your code.
Gamemaker's Debug Log
Reading Gamemaker's debug log can be helpful in telling you what's wrong with your code. This usually shows up when there's an issue compiling your code, and Gamemaker will tell you which lines of code are causing this issue. In this example, the code wouldn't compile in the 'Left Pressed' method in my object 'Object2' because the variable 'bounce' doesn't exist.
It's helpful to copy this debug log before you abort so you can reference it while fixing your code.
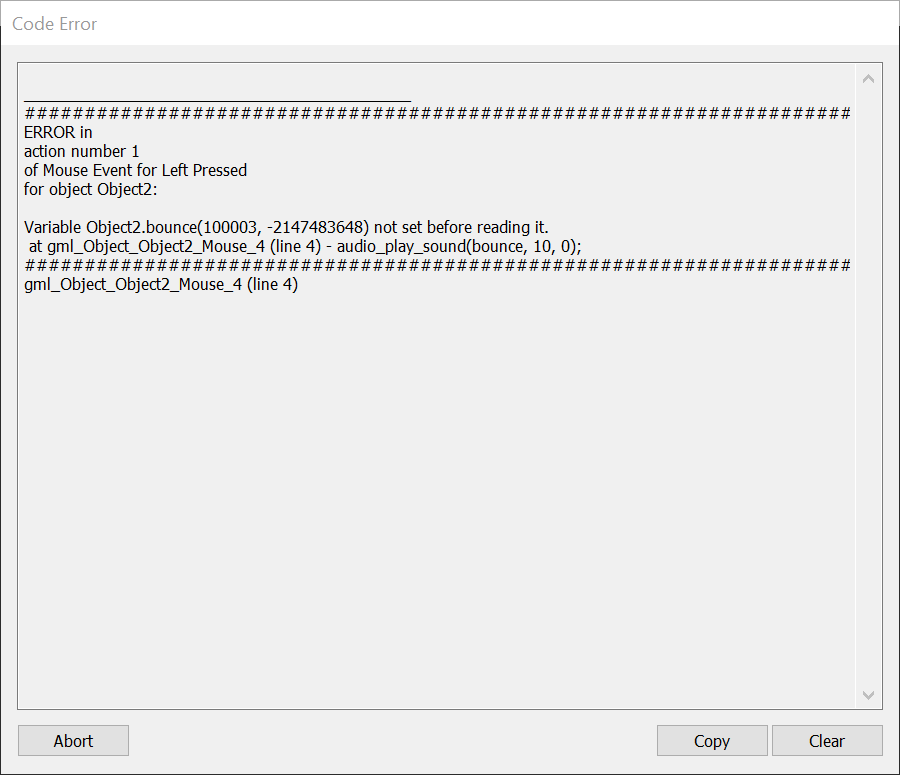
When in doubt, Google it
If you ever run into a bug and you can't figure out how to fix it, researching solutions online can be very helpful. There are lots of resources online to help you, such as:
Gamemaker Community: https://forum.yoyogames.com/index.php
Gamemaker Manual: https://manual.yoyogames.com/#t=Content.htm
Stack Overflow: https://stackoverflow.com/questions/tagged/game-maker
r/gamemaker: https://www.reddit.com/r/gamemaker/
We encourage you to use these resources for debugging, but as a reminder, DO NOT COPY CODE. Not only is it a violation of academic integrity, but it will hold you back when learning how to program in Gamemaker.
As always, we are here to help you. Feel free to contact the TA's through discord and visit our office hours if you ever need help with your code.
ASU Game Certificate Devlog
More posts
- A Guide to Writing a Good Rules DocumentFeb 02, 2024
- How to Write a Sample Play DocumentFeb 02, 2024
- Procedural Generation vs Random GenerationOct 21, 2022
- Introduction to State MachinesOct 07, 2022
- Tips for Writing a Good Pitch DocumentSep 23, 2022
- Integrating Github with Gamemaker StudioSep 02, 2022
Leave a comment
Log in with itch.io to leave a comment.